JSonWebToken Passthrough
Describes how to authenticate a user on your site with MedChat
Setting up authentication passthrough on the MedChat Widget involves three steps.
- Generate a shared secret from the Developer page (Admin -> Developer).
- Create an endpoint on your system to generate a signed JSON Web Token (JWT) using the shared secret.
- Use the endpoint on the page(s) that will contain the MedChat widget and pass the resulting JWT to the widget.
Shared Secret Creation
To create a shared secret goto the Developer page (Admin -> Developer). In the API tab, click the Generate new key button and follow the dialog to generate the key.
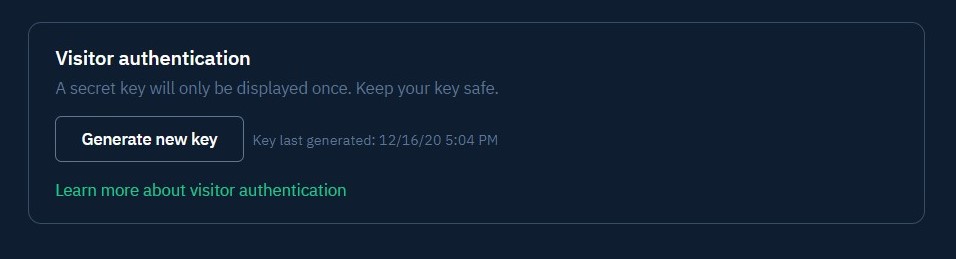
Generate secret key dialog
Remember to keep the generated value secure
Jwt Creation Endpoint
An API endpoint must be created on your system that generates and returns a signed JWT using the HMAC SHA256 algorithm in order to securely identify a user on your system. The payload of the JWT must have the following values:
Name | Required | Description |
---|---|---|
name | Yes | User’s Name. Max length 200 characters |
No | User’s Email. Max length 200 characters. | |
external_id | Yes | Alphanumeric string that uniquely identifies the user. Max length 200 characters |
iat | Yes | Indicates the UTC date/time when the JWT was issued. POSIX time format in seconds from Epoch. Exp: 1592413831 See: https://www.epochconverter.com/ |
exp | Yes | Indicates when this jwt will expire in UTC. Should be in POSIX time format in seconds from Epoch. See: https://www.epochconverter.com/ |
Below is an example for creating a JWT using c#, and the JsonWebToken nuget package.
public static string GenerateExternalUserVerificationToken(string sharedSecret, string externalUserId, string name, string email, DateTime? issuedDateTime = null, DateTime? expiredDateTime = null)
{
issuedDateTime = issuedDateTime ?? DateTime.UtcNow;
expiredDateTime = expiredDateTime ?? DateTime.UtcNow.AddHours(4);
var symmetricJwk = SymmetricJwk.FromBase64Url(sharedSecret);
var payload = new JwtObject();
payload.Add("name", name);
payload.Add("email", email);
payload.Add("external_id", externalUserId);
var descriptor = new JwsDescriptor(new JwtObject(), payload)
{
SigningKey = symmetricJwk,
Algorithm = SignatureAlgorithm.HmacSha256,
IssuedAt = issuedDateTime,
ExpirationTime = expiredDateTime,
};
var writer = new JwtWriter();
return writer.WriteTokenString(descriptor);
}
The endpoint should be secure so that a JWT can not be created by an anonymous user. For more about JWTs and libraries for specific languages, see https://jwt.io/.
Widget API
In addition to the necessary API endpoint creation to serve user JWT tokens, client-side code must be added to register the returned user token with the widget. After the API endpoint is called to grab the JWT token, the client-side code must call the MedChat Widget API function registerJWT(), passing in the JWT, in order for the widget to utilize the validation.
The below pseudo-code illustrates the general flow of what that client-side code would look like on your page that contains the widget instance:
// Logged in user detected
// url for api endpoint serving is stored in var url
fetch(url)
.then(response => response.json())
.then(resp => {
// pass JWT token into MedChat Widget API registerJWT function
window.medchatapp.registerJWT(resp.token);
})
.catch(error => {
console.log(error);
});
The MedChat Widget API also provides a mechanism to un-verify users when your client system detects a user has logged out. When that scenario is detected in your client side call, a user can be un-verified with something similar to the pseudocode below.
// User Log out detected on the page with the widget
...
.then(() => window.medchatapp.unVerifyUser());
After this function is called, subsequent messages sent from the widget instance, for any ongoing chats, will no longer be marked verified.
Using Verified User Name in Widget Greeting Message
Once the widget authentication passthrough mechanism has been setup & instrumented, you are able to utilize the verified user's name inside of your widget greeting message.
Inserting the variable {{verified-user.verifiedName}}, including the surrounding {{}}, inside your widget greeting message will enable this feature. Then when the widget is rendered in the browser the variable will be replaced with the authenticated user's name, if authenticated, otherwise it will replace the variable with an empty string.
Updated over 2 years ago